Laravel is very popular PHP framework today and Laravel 5.3 released few days ago. There are several changes and enhancement on Laravel 5.3 version.
So, Today i want to share with you how to image uploading in Laravel 5.3 with validation. If you are beginners then you can do that simply by following few step. Laravel 5.3 provide very simple way to create file uploading with proper validation like max file size 2MB, valid file extension like jpeg,png,jpg,gif or svg etc. So you can easily also implement this on your laravel 5.3 application.
I give you very easy and simple example that way you can understand very well. So you have to just following few step and get output like as bellow preview:
Preview:
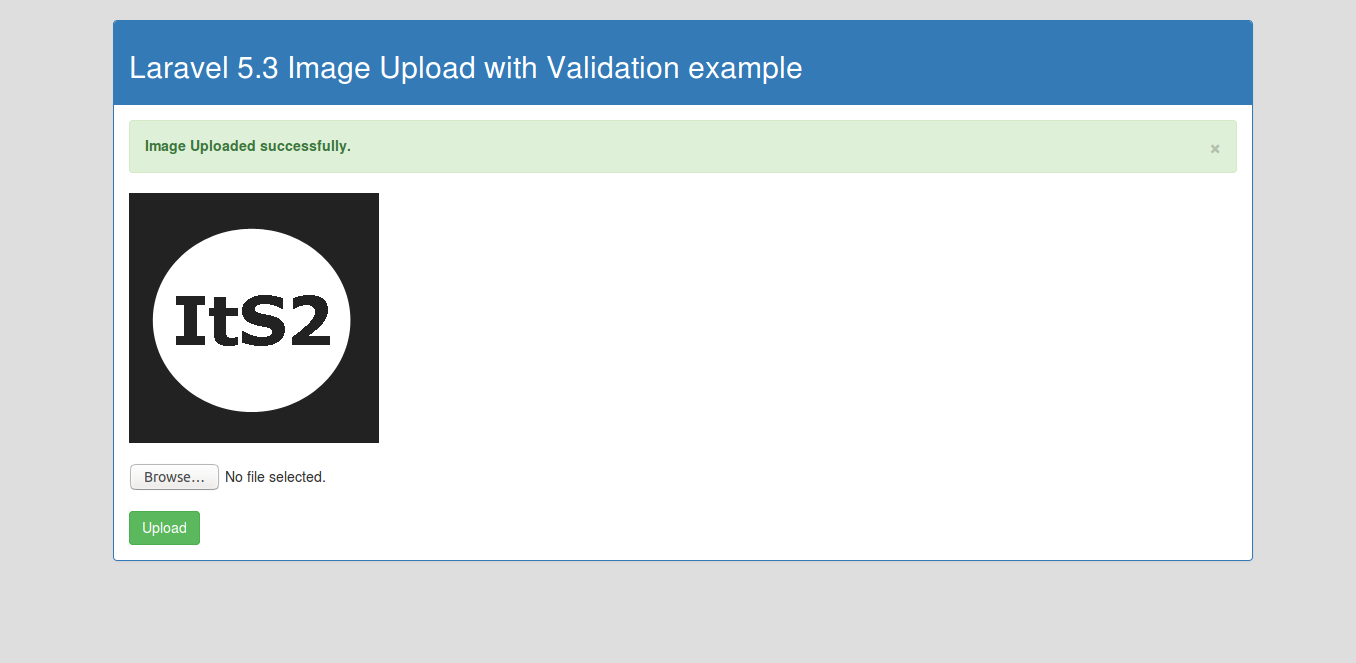
Step 1: Add Route
First we have add two route in routes.php file. first one for generate view and second one for post method. so let's add bellow route in your route file.
routes/web.php
Route::get('image-upload','ImageController@imageUpload');
Route::post('image-upload','ImageController@imageUploadPost');
Step 2: Add Controller
Ok, now we need to create ImageController.php file. If you don't have ImageController then you can create new and put bellow code on that file. Make sure you have "images" folder with full permission in your public directory. we will store image in images directory.
app/Http/Controllers/ImageController.php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Http\Requests;
class ImageController extends Controller
{
/**
* Create view file
*
* @return void
*/
public function imageUpload()
{
return view('image-upload');
}
/**
* Manage Post Request
*
* @return void
*/
public function imageUploadPost(Request $request)
{
$this->validate($request, [
'image' => 'required|image|mimes:jpeg,png,jpg,gif,svg|max:2048',
]);
$imageName = time().'.'.$request->image->getClientOriginalExtension();
$request->image->move(public_path('images'), $imageName);
return back()
->with('success','Image Uploaded successfully.')
->with('path',$imageName);
}
}
Step 3: Create Blade File
In At Last we require to create view file for image or file uploading. so you can create image-upload.blade.php and put following code in that file.
resources/views/image-upload.blade.php
<!DOCTYPE html>
<html>
<head>
<title>Laravel 5.3 Image Upload with Validation example</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css">
</head>
<body>
<div class="container">
<div class="panel panel-primary">
<div class="panel-heading"><h2>Laravel 5.3 Image Upload with Validation example</h2></div>
<div class="panel-body">
@if (count($errors) > 0)
<div class="alert alert-danger">
<strong>Whoops!</strong> There were some problems with your input.<br><br>
<ul>
@foreach ($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
</div>
@endif
@if ($message = Session::get('success'))
<div class="alert alert-success alert-block">
<button type="button" class="close" data-dismiss="alert">×</button>
<strong>{{ $message }}</strong>
</div>
<img src="/images/{{ Session::get('path') }}">
@endif
<form action="{{ url('image-upload') }}" enctype="multipart/form-data" method="POST">
{{ csrf_field() }}
<div class="row">
<div class="col-md-12">
<input type="file" name="image" />
</div>
<div class="col-md-12">
<button type="submit" class="btn btn-success">Upload</button>
</div>
</div>
</form>
</div>
</div>
</div>
</body>
</html>
来自 http://itsolutionstuff.com/post/laravel-53-image-upload-with-validation-exampleexample.html
Laravel 5.3 - image dimension validation rules example
By Hardik Savani | September 24, 2016 | | 45457 Viewer | Category : Laravel
Laravel 5 provide new image dimensions validation option for image upload. In this validation rules we can set several rules like as listed bellow:
Dimensions Rules:
1)width
2)height
3)min_width
4)min_height
5)max_width
6)max_height
7)ratio
In this Dimensions option through we can set fix width and height, if invalid width and height of image then it will return error. Same way we can set validation min and max height width on your validation.
Few days ago i posted image upload with validation post in Laravel 5.3, you can see here : Laravel 5.3 Image Upload with Validation example.
In this post i used simple validation with mime type and max size like as bellow :
$this->validate($request, [
'image' => 'required|image|mimes:jpeg,png,jpg,gif,svg|max:2048',
]);
You can also replace your validation using Dimensions rules like as bellow:
Example 1:
$this->validate($request, [
'image' => 'required|image|mimes:jpeg,png,jpg,gif,svg|max:2048|dimensions:width=500,height=500',
]);
Example 2:
$this->validate($request, [
'image' => 'required|image|mimes:jpeg,png,jpg,gif,svg|max:2048|dimensions:min_width=350,min_height=600',
]);
Example 3:
$this->validate($request, [
'image' => 'required|image|mimes:jpeg,png,jpg,gif,svg|max:2048|dimensions:max_width=350,max_height=600',
]);
Example 4:
$this->validate($request, [
'image' => 'required|image|mimes:jpeg,png,jpg,gif,svg|max:2048|dimensions:ratio=3/2',
]);
来自 https://itsolutionstuff.com/post/laravel-53-image-dimension-validation-rules-exampleexample.html
来自 https://laracasts.com/discuss/channels/general-discussion/image-validation
max:200
instead? – Marc B Apr 6 '15 at 18:42