laravel5 数据库配置(MySQL)
首先有一个安装完成可以运行的laravel框架。
配置database.php
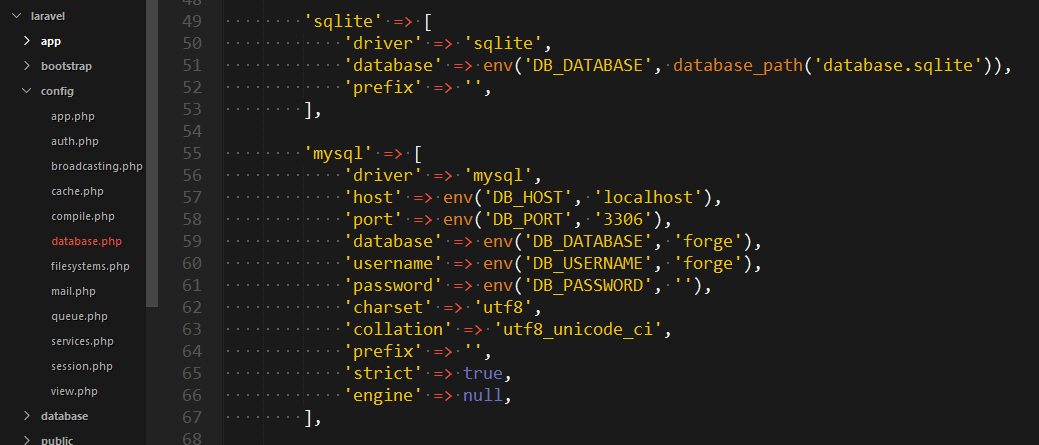
'mysql' => [ 'driver' => 'mysql', //数据库的类型
'host' => env('DB_HOST', 'localhost'), //数据库的位置
'port' => env('DB_PORT', '3306'), //端口号
'database' => env('DB_DATABASE', 'forge'), //数据库名
'username' => env('DB_USERNAME', 'forge'), //用户名
'password' => env('DB_PASSWORD', ''), //密码
'charset' => 'utf8', //字符集
'collation' => 'utf8_unicode_ci', //排序方式
'prefix' => '', //前缀
'strict' => true, //Strict模式
'engine' => null, //引擎],
'engine' => null, //引擎
MySQL部分代码如上。
我的修改如下:
'mysql' => [ 'driver' => 'mysql', 'host' => env('DB_HOST', 'localhost'), 'port' => env('DB_PORT', '3306'), 'database' => env('DB_DATABASE', 'mydb'), //这里是我的数据库名
'username' => env('DB_USERNAME', 'root'), //这里是用户
'password' => env('DB_PASSWORD', ''), //密码
'charset' => 'utf8', 'collation' => 'utf8_unicode_ci', 'prefix' => '', 'strict' => true, 'engine' => null,
],
修改.env
配置完database.php后,进行.env的配置。
.env是框架的环境变量,是为了让这个选项在不同环境下有不同的值。
.env文件在laravel根目录下。
只需要对文件的数据库部分进行修改。
DB_CONNECTION=mysqlDB_HOST=[数据库地址]DB_PORT=[端口(3306)]DB_DATABASE=[数据库]DB_USERNAME=[用户名]DB_PASSWORD=[密码]
我的修改如下:
DB_CONNECTION=mysqlDB_HOST=127.0.0.1DB_PORT=3306DB_DATABASE=mydbDB_USERNAME=rootDB_PASSWORD=
创建数据表
我这里建立一个名为user的迁移
注:要在框架的根目录下。
在cmd中执行:
php artisan make:migration create_user_table
运行成功如下图

这样我们就可以在database/migrations目录下发现我们新生成的文件。
2016_09_20_123557_create_user_table.php
文件名前一部分是建立的时间后一部分是执行的名称。
文件如下:
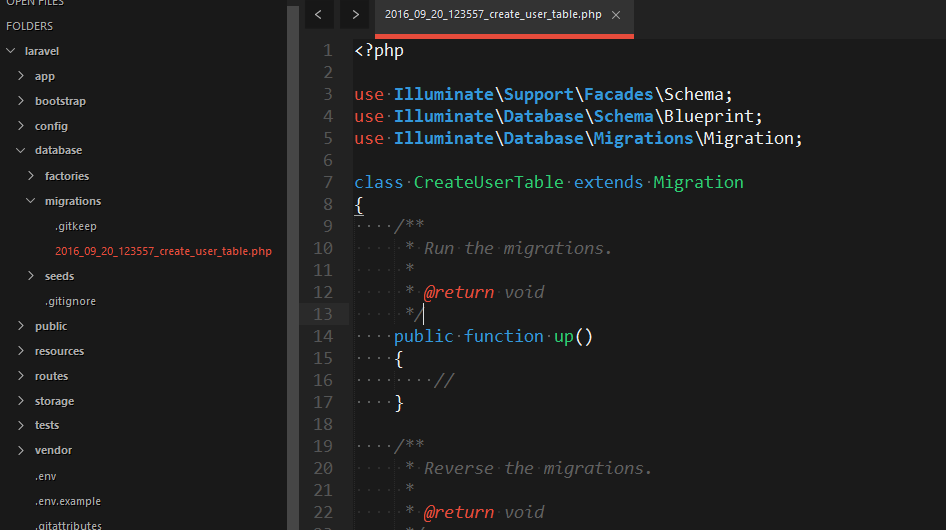
<?php
use Illuminate\Support\Facades\Schema;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Database\Migrations\Migration;class CreateUserTable extends Migration{ /**
* Run the migrations.
*
* @return void
*/
public function up()
{ //这里是新增消息(表,列,索引)的位置
} /**
* Reverse the migrations.
*
* @return void
*/
public function down()
{ //这里删除信息的位置
}
}
public function up()
{
Schema::create('user', function (Blueprint $table) { //建立数据表user
$table->increments('id'); //主键自增
$table->string('name')->unique(); //'name'列唯一
$table->string('password'); //'password'
$table->string('email')->unique(); //'email'唯一
$table->timestamps(); //自动生成时间戳记录创建更新时间
});
}
接下来。
在cmd中执行
php artisan migrate
成功后如图:

则表明建表成功。
进入数据库可以看见表。
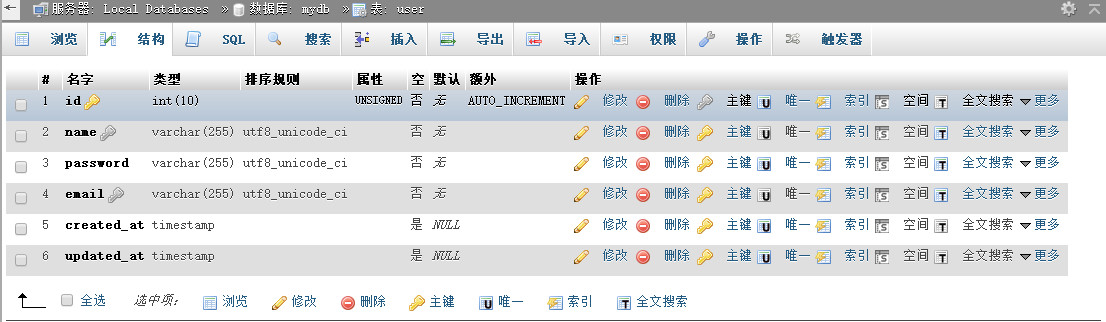
这样数据库的基本配置部分就完成了。